Logging In and Activating a QMENTA Project¶
Log in¶
Note
Prior to using the QMENTA Client API, all users must be registered to the QMENTA Platform.
In order to log in, establish a connection with the QMENTA Platform by creating an instance of your account using your username and password:
import qmenta.client
# Create a connection (logs in automatically).
account = qmenta.client.Account("user", "password")
# This will generate a request for your user, password, and two-factor authentication (if enabled).
Once logged in, you can check your available Projects as shown next:
print(account.projects)
Example output:
[{'id': 80, 'name': 'Nunc interdum lacus sit'},
{'id': 43, 'name': 'Test Project'}]
See that each element in the list is composed of a Project ID (id
) and a Project Name (name
).
Project ID is the unique identifier of the Project.
Note
The QMENTA Platform support various types of Projects and user roles. Having a restricted role may limit the functionalities of this API. See Roles and Permissions for further details.
Activate a Project¶
To activate a Project, its Project ID is required. Identify the Project ID as shown above, via the Project Name, or directly from the QMENTA Platform dashboard as shown in the following image.
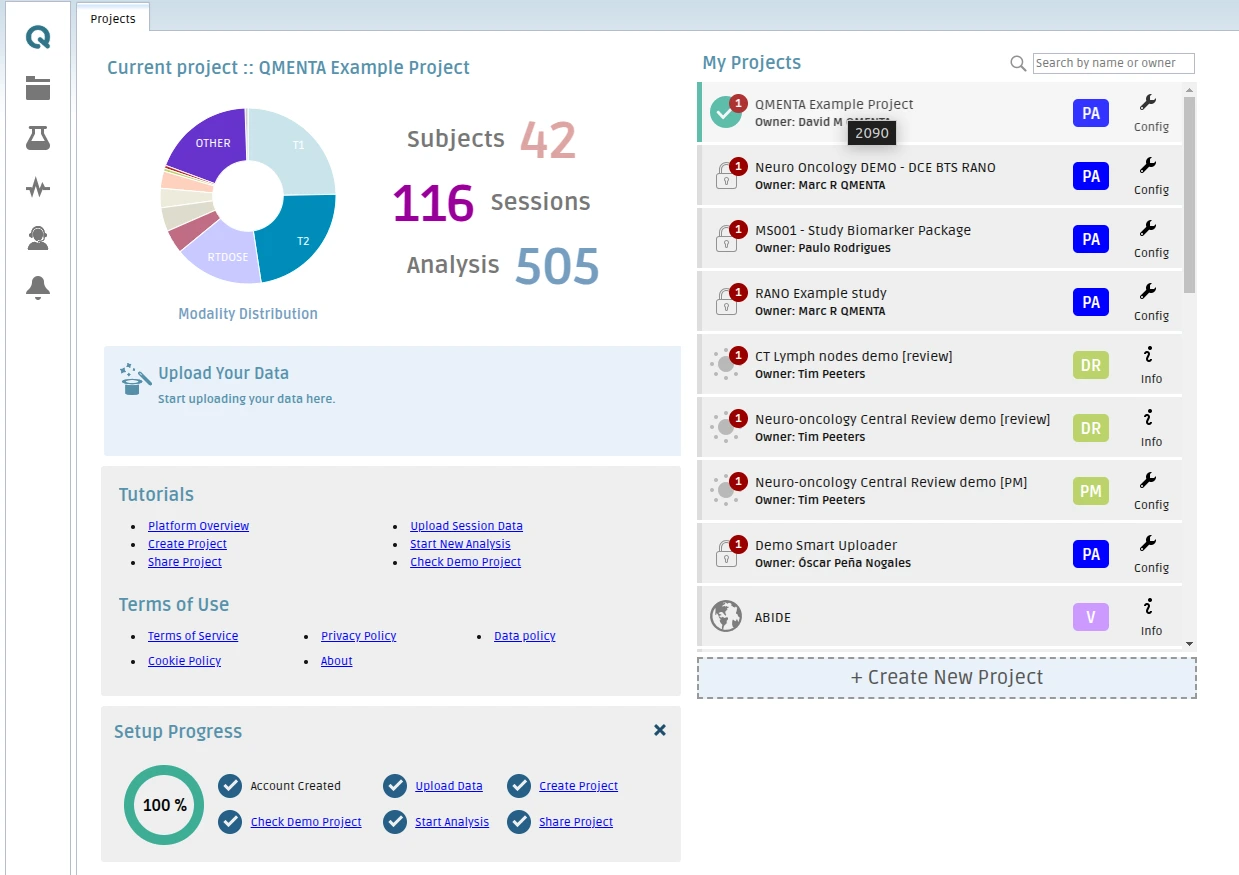
In the QMENTA Platform dashboard you can obtain the Project ID by hovering the mouse over the desired Project.¶
Activate a Project using its Project ID as follows:
# Define Project ID.
project_id = "43"
# Activate Project
project = qmenta.client.Project(account, project_id)
Activate a Project indirectly via its Project Name as follows:
# Retrieve the Project ID using the Project Name:
project_name = "Test Project"
project = next((qmenta.client.Project(account, p["id"]) for p in account.projects if p["name"] == project_name), None)
The variable project
is an instance of Project
, which allows you to interact with the Project’s data and settings.
Next Steps¶
Explore the following sections in detail:
Data Handling – Upload data.
Data Searches – Perform data and metadata searches.
Metadata Handling – Modify metadata.
Analyses Execution – Execute analyses.
Results Handling – Retrieve and store analysis outputs.