Tutorial: QMENTA Client APIΒΆ
This tutorial will guide you through the basic steps of using the QMENTA API, from logging into your account to managing your available QMENTA Projects. Once you have access to a QMENTA Project, you can perform the actions as explained in the following sections of this tutorial.
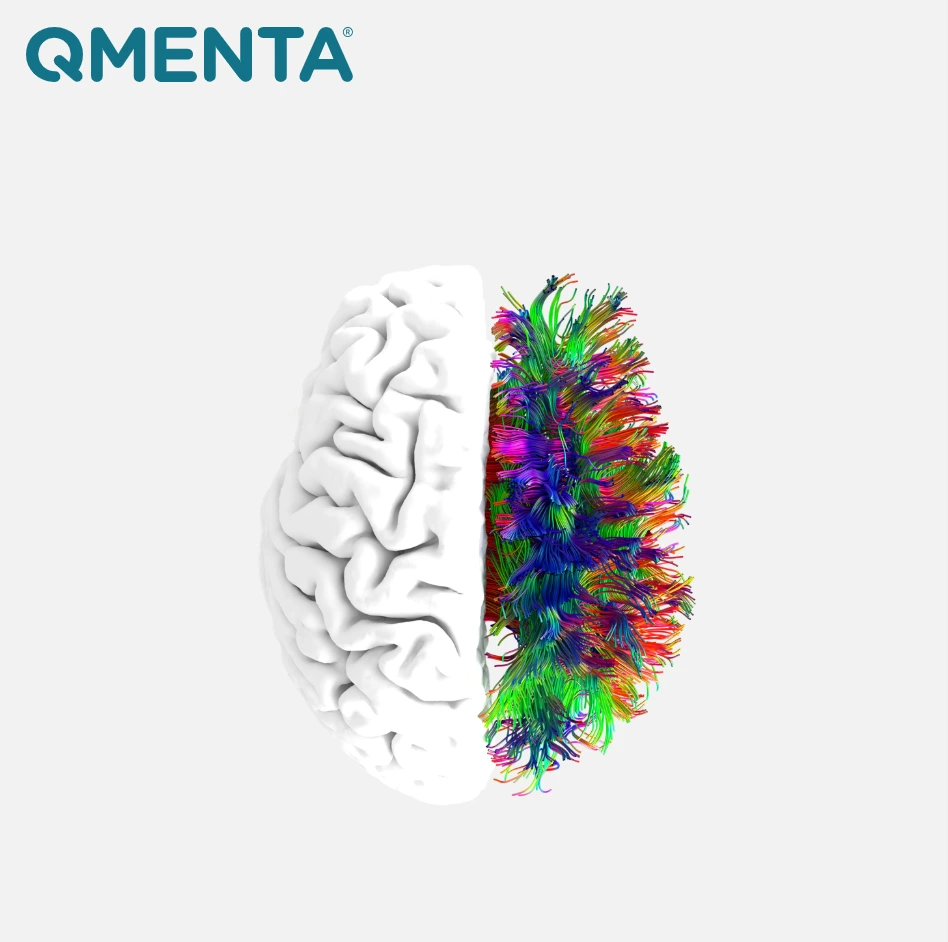
Note
For a greater understanding of this API tutorial, it is strongly recommended to be familiar with the QMENTA Platform and the QMENTA User Manual.
Tutorial Sections
Each of these functionalities will be covered in detail in their respective sections.